A Complete Guide to JavaScript Application Development
- Leanware Editorial Team
- Sep 24, 2024
- 9 min read
Updated: Sep 25, 2024
JavaScript has evolved quite a bit from its roots as an unassuming scripting language for web browsers. These days, it's the full-stack workhorse of choice for nearly every type of application imaginable. Whether you've ever tried building up an app or want to try your hands on one, whether it's web App / mobile App or even Desktop software JavaScript has everything set for you.
Let's see how we can approach the development of a JavaScript application, from the very basics to advanced techniques, to enable you to develop the applications you really want to create with confidence.
What Is JavaScript and Why Is It Important?
JavaScript is a lightweight, high-level programming language that's primarily known for its role in web development. It allows developers to add interactivity and dynamic behavior to websites, making them more engaging and user-friendly.
The importance of JavaScript in modern development can't be overstated. It's:
It runs on virtually every device with a web browser.
It can be used for front-end, back-end, and full-stack development.
Modern JavaScript engines are highly optimized for performance.
Supported by a vast ecosystem - libraries, frameworks, and tools available.
Can I Do App Development with JavaScript?
Yes, JavaScript can create fully functional applications. With Node.js, which allows JavaScript to run on the server side, and frameworks like Electron for desktop apps, JavaScript has become a full-stack solution for application development.
These are some types of applications you can build with JavaScript:
Web Applications: Using frameworks like React, Vue, or Angular.
Mobile Apps: With frameworks like React Native or Ionic.
Desktop Apps: Using Electron or NW.js.
Server-side Applications: With Node.js and frameworks like Express or Koa.
Progressive Web Apps (PWAs): Combining web and mobile app features.
What Is JavaScript in Web Application Development?
In web application development, JavaScript plays a more significant role in creating interactive and dynamic user experiences. It allows developers to:
Manipulate the Document Object Model (DOM)
Handle user events (clicks, input, etc.)
Make asynchronous requests to servers (AJAX)
Update content without refreshing the page
Implement complex UI components and animations
Modern web applications use JavaScript frameworks like React, Vue, and Angular to build complex single-page applications (SPAs) that help render a smooth, app-like experience in the browser.
Why Use JavaScript for Mobile Apps Development?
JavaScript has become a popular choice for mobile app development. And, no doubt it is due to several factors:
Cross-platform development: Write once, run on both iOS and Android.
Faster development cycles: Leverage existing web development skills.
Large community and ecosystem: Access to numerous libraries and tools.
Performance improvements: Modern frameworks offer near-native performance.
Cost-effective: Reduce development time and resources.
React Native and Ionic have made it super easy to build great mobile apps with JavaScript. You can now use the same web development skills you already know to create mobile apps, making the whole process feel a lot more natural.
Popular Frameworks for JavaScript App Development
The JavaScript ecosystem is rich with frameworks and libraries that can greatly speed up your development process.
These are some popular options you can choose from:
React: A library for building user interfaces, widely used for web and mobile (React Native) development.
Vue.js: A progressive framework for building UIs, known for its simplicity and flexibility.
Angular: A comprehensive framework for building large-scale web applications.
Express.js: A minimal and flexible Node.js web application framework.
Electron: A framework for creating native applications with web technologies.
Node.js: A JavaScript runtime built on Chrome's V8 JavaScript engine.
Each framework offers something different, making them better for certain projects.
It’s just about choosing the right JavaScript frameworks for web and app development that work best for you.
Choosing the Right Framework for Your Project
Choosing the right framework really depends on a few key things:
Your project’s needs: What’s the scale and complexity?
Performance: Some frameworks handle certain apps better than others.
Team’s skillset: How familiar is your team, and how much time do you have to learn?
Community support: Bigger communities can mean more resources and solutions.
Long-term stability: Make sure it’s something that will be maintained.
Don't go for what's trendy; choose the framework that will fit your project and your team.
Building Your First JavaScript Application
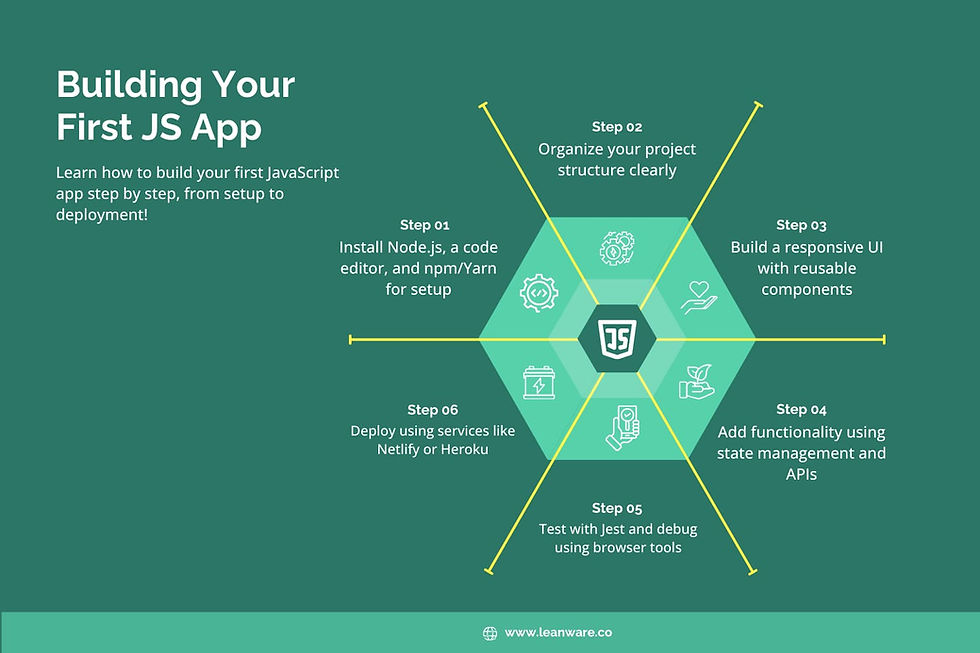
1. Setting Up Your Development Environment
To get started with JavaScript app development, you’ll first need to set up your development environment. Here's a quick overview of what you'll need:
Install Node.js: Download and install from nodejs.org.
Choose a code editor: Popular options include Visual Studio Code, WebStorm, or Sublime Text.
Set up version control: Install Git and create a GitHub account.
Install a package manager: npm (comes with Node.js) or Yarn.
For more advanced setups, consider:
2. Creating the Project Structure
Having a clear project structure makes everything easier to manage and maintain in the long run. Here's a basic structure for a web application:
Adjust this structure based on your project's needs and the framework you're using.
3. Developing the User Interface
When developing the UI, consider these best practices:
Use a component-based architecture for reusability.
Implement responsive design for various screen sizes.
Follow accessibility guidelines to ensure your app is usable by everyone.
Use CSS-in-JS or a preprocessor like Sass for more maintainable styles.
Here's a simple example of a React component:
4. Adding Dynamic Functionality with JavaScript
JavaScript brings your application to life. Here are some key areas to focus on:
State management: Use tools like Redux or MobX for complex applications.
API integration: Fetch data from backend services using the Fetch API or Axios.
Event handling: Respond to user interactions effectively.
Performance optimization: Implement lazy loading and code splitting.
Example of fetching data in a React component:
5. Testing and Debugging Your Application
Testing is also important for ensuring the reliability of your application. Here are some testing strategies:
Unit testing: Test individual components and functions.
Integration testing: Test how components work together.
End-to-end testing: Test the entire application flow.
Try using Jest for unit tests and Cypress for end-to-end tests. They’re both reliable tools that can help you ensure everything works smoothly as you build your app.
For debugging, here are some useful tips:
Use your browser’s developer tools to handle client-side issues.
Add logging throughout your app to track what’s happening.
Take advantage of the debugging tools built right into your IDE.
These steps can really help you spot and fix bugs quickly while working on your project.
6. Deploying Your Application
Deployment options depend on what type of app you’re building:
Web apps: Use services like Netlify, Vercel, or AWS Amplify for static sites, and Heroku or DigitalOcean for full-stack apps.
Mobile apps: Distribute through the App Store for iOS or Google Play for Android.
Desktop apps: Share via your website or platforms like Steam.
For a smoother process, consider setting up a CI/CD pipeline to automate testing and deployment.
Understanding Web and Mobile Application Architecture
When moving from simple web projects to full application development, it’s important to get familiar with a few key concepts:
How client-server architecture works
Designing RESTful APIs
Managing complex state
Handling authentication and security
Caching and optimizing performance
For mobile apps, also think about:
The pros and cons of native vs hybrid development
Mobile-specific UI/UX
Offline support and syncing data
Managing push notifications and background tasks
Making Build and Deployment Decisions
As your app grows, you'll face important decisions around how to handle building and deployment. You can start by choosing the right build tools like Webpack or Rollup to manage your assets efficiently.
Next, improve performance by implementing code splitting. Make sure to set up environment-specific configurations to keep development smooth.
Automating your build process with CI/CD pipelines is a huge time-saver. Plus, adding monitoring and error tracking helps catch any production issues early on.
Tools like GitHub Actions or GitLab CI make automating both building and deploying your app much easier and more efficient.
Should I Learn JavaScript for App Development?
There are numerous reasons why you should learn JavaScript for building apps. As a language, it is quite versatile so you can use it in front-end, back-end, and mobile development itself as well.
And the demand for JavaScript developers is extraordinarily high - not to mention all of the libraries and tools in the ecosystem that are built to help you do your job well.
JavaScript has a strong, active community to help when needed, you’ll have access to new tools and features that keep your skills up-to-date and your apps competitive.
Essential Skills for JavaScript Developers
To get really good at JavaScript app development, make sure to focus on these key areas:
Core JavaScript concepts (closures, prototypes, async programming)
ES6+ features (arrow functions, destructuring, modules)
DOM manipulation and event handling
Asynchronous programming (Promises, async/await)
HTTP and RESTful API concepts
State management techniques
Performance optimization
Testing methodologies
Version control with Git
Familiarity with build tools and package managers
Practice Projects to Build Your Skills
To really develop your skills with JavaScript, you can begin with a small project.
You could start off creating something like a To-Do List app to get a handle on just the basic concepts of CRUD or a simple weather app to learn how to work with APIs.
If you want it even tougher, build an E-commerce site to work on user authentication and state management.
You could also try doing a real-time chat app to learn about WebSockets or clone a mobile app for hands-on mobile development. Keep your code clean and adopt best practices as you go.
Is Python Better than JavaScript for App Development?
Python is great for app development and JavaScript too! JavaScript balances this out by being reasonably accessible via the web browser, having a pretty solid front-end ecosystem built around it, as well as being performant for real-time apps.
Python, on the other hand, keeps things clean and simple - a general-purpose language that is good in readability with dedicated libraries in backend development to data analysis to machine learning using Jupyter Notebooks.
This decision depends on your project requirements and your developer preference.
JavaScript vs. Python: Key Differences for Developers
Aspect | JavaScript | Python |
Syntax | Uses curly braces {} and semicolons ; | Uses indentation for structure, no braces or semicolons |
Typing | Dynamically typed | Dynamically typed with optional static typing (using typing) |
Concurrency | Event loop model (async programming) | Multiple concurrency models (e.g., threading, asyncio) |
Use cases | Web dev, mobile, and desktop applications | Scientific computing, data analysis, and backend |
Learning curve | Moderate, with more syntax complexity | Easier for beginners due to simple, readable syntax |
Some developers teach themselves both, and they prove invaluable in efficiently completing a broad variety of projects.
Ensuring Performance and Security
To improve the efficiency, we better start using code splitting and lazy loading to only load resources when needed. Server-side rendering can improve the initial load time and you should also use compression and minify to optimize your assets.
Check that your state management in app is not very slow. If you have a lot of complex calculations, that are heavy on CPU, then consider using Web Workers to offload the main thread and deliver a better experience.
Security Best Practices
These fundamental things to comply with to secure your app:
Implement proper authentication and authorization
Use HTTPS for all communications
Sanitize user inputs to prevent XSS attacks
Implement Content Security Policy (CSP)
Keep dependencies updated to patch vulnerabilities
Best Practices for Cross-Platform Compatibility
When developing cross-platform apps, remember to implement responsive design so it looks great on any device.
Beware of platform-specific APIs and always test for multiple device/browser combos. It ensures that your app will still work under difficult circumstances by implementing progressive enhancement strategies.
Alternatively, you may just consider using a framework like React Native or Ionic to write the code once and also run it on different platforms. It gives a more consistent and standardized approach.
Continuous Improvement and Updating Your Applications
In order to maintain the relevance and security of your applications:
Maintain up-to-date dependencies and frameworks.
Re-write code to make it more maintainable
Use automated testing to catch regressions
Accept user feedback and act on it
Learn more about new JavaScript features and best practices
Feature flags are a great way to release new features while controlling the percentage of users that are exposed and A/B testing will enable you to measure user experience.
Wrapping Up
With JavaScript application development, you'll be creating some very powerful dynamic web apps, as well as very innovative and powerful mobile solutions. Its flexibility makes it the first choice for all the modern, efficient, cross-platform projects of these days.
So be curious, keep learning, and keep experimenting with new tools. Whether just entering or leveling up, JavaScript provides a solid base to create innovative, scalable apps.
For developers who prefer programming in statically and strongly typed languages, there is a superset of JavaScript called TypeScript, which is highly adopted by the industry.
For this reason, if ever you need expert advice or resources towards furthering your development journey, take a peek at what Leanware has up its sleeve.
Happy coding!