As software developers, we're accustomed to thinking in terms of binary states - true or false, 0 or 1. However, quantum computing introduces a fundamentally different paradigm that reshapes how we approach software development.
In this comprehensive guide, we'll explore what quantum computing means for developers and how to prepare for this technological shift.
TL;DR
Quantum computing's qubits process multiple states simultaneously, threatening current cryptography. Quantum computers with 4,099 qubits could break RSA encryption in ~10 hours. Developers should document cryptographic assets, implement quantum-resistant algorithms like CRYSTALS-Kyber, and use hybrid approaches. Begin quantum-safe transition now using Open Quantum Safe Project and NIST guidelines.
Basics of Quantum Computing
To understand quantum computing's implications for software development, let's examine its core concepts through familiar programming analogies.
What Are Qubits?
Think of a traditional bit in your code. When you declare a boolean variable, it can only be true or false:
Traditional bit:
A qubit (quantum bit) operates differently. Instead of being limited to one state, it can exist in multiple states simultaneously until observed. Imagine a variable that could process both true AND false at the same time:
A qubit (quantum bit) is the quantum equivalent of a classical bit but with a difference. While a classical bit must be either 0 or 1, a qubit can exist in a combination of both states at once - a property called superposition.
The power of qubits comes from this ability to exist in multiple states. With traditional bits, adding one bit doubles your possible states. With qubits, each additional qubit exponentially increases your computational possibilities. Three classical bits can represent one of eight possible values at a time. Three qubits can process all eight values simultaneously.
1 classical bit: 2 possible states (0 or 1)
2 classical bits: 4 possible states (00, 01, 10, 11)
3 classical bits: 8 possible states
But with qubits:
1 qubit: Can process 2 states simultaneously
2 qubits: Can process 4 states simultaneously
3 qubits: Can process all 8 states simultaneously
Key Principles: Superposition and Entanglement
Two fundamental principles make quantum computing powerful:
Superposition
Remember parallel processing in traditional computing? Superposition takes this to an entirely new level. Instead of running multiple processes in parallel, a quantum computer can process multiple states within a single qubit. This isn't just doing things faster - it's a fundamentally different way of processing information.
Consider a traditional array search:
A quantum algorithm, using superposition, could theoretically check all elements simultaneously - not just faster, but in a fundamentally different way.
Entanglement
This is where quantum computing gets even more interesting. Entanglement means two or more qubits can be correlated in ways that have no classical equivalent. When qubits are entangled, the state of one instantly affects the others, regardless of distance.
Think of it like this: In traditional programming, if you have two variables:
With entangled qubits, changing one automatically and instantly affects the other. This property enables quantum computers to solve certain problems exponentially faster than classical computers.
Quantum Computing's Impact on Cryptography
Quantum computing greatly challenges current cryptographic systems through its ability to solve previously intractable mathematical problems. This fundamental shift affects two critical areas:
How Shor's Algorithm Affects Encryption
Most current encryption systems rely on the mathematical difficulty of factoring large numbers. For example, RSA encryption uses the fact that while multiplying two large prime numbers is easy, finding those original prime numbers from their product is extremely difficult for classical computers.
Shor's algorithm, running on a quantum computer, can factor large numbers exponentially faster than classical computers. This isn't just a speed improvement - it fundamentally breaks the mathematical problem that makes current encryption secure.
Weaknesses in Current Encryption
RSA-2048
RSA encryption, widely used in secure communications, relies on the mathematical difficulty of factoring large numbers. Let's understand why RSA-2048 is vulnerable to quantum attacks:
Current RSA-2048 security relies on this principle:
Classical computer time to break: Billions of years
Quantum computer (with sufficient qubits): Hours
This isn't science fiction - IBM estimates that a quantum computer with 4,099 logical qubits could break RSA-2048 in just 10 hours. While current quantum computers haven't reached this capability, development is progressing rapidly.
Effects on SHA-256
SHA-256, commonly used in blockchain technology and password hashing, shows more resilience against quantum attacks but isn't entirely safe. Here's why:
Current Security Level:
Classical security: 256 bits
Quantum security: Effectively 128 bits (due to Grover's algorithm)
This means that while quantum computers don't immediately break SHA-256, its security is significantly reduced. Applications using SHA-256 will need to either:
Increase key sizes
Switch to quantum-resistant alternatives
Implement hybrid solutions
Post-Quantum Cryptography
Post-quantum cryptography (PQC) focuses on developing cryptographic algorithms that withstand attacks from both classical and quantum computers. For developers, this means implementing new mathematical approaches to protect data and communications against future quantum threats.
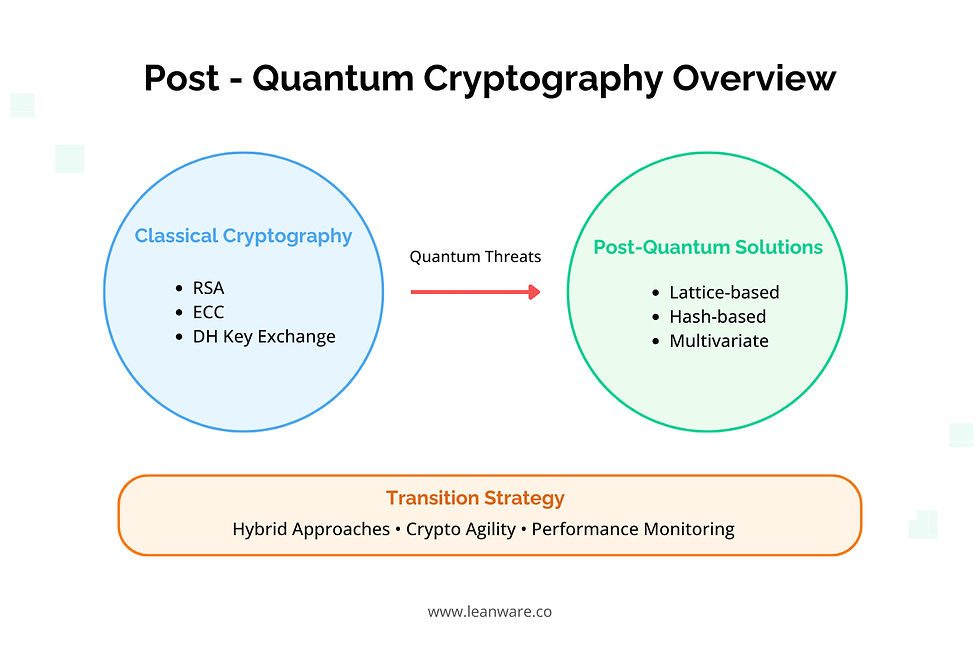
What Is It?
Unlike current cryptographic methods that rely on factoring large numbers or solving discrete logarithms, PQC uses mathematical problems that remain complex even for quantum computers. These include lattice-based problems, multivariate equations, and hash-based signatures.
This shift in mathematical foundations ensures your cryptographic implementations remain secure even when powerful quantum computers become available.
How to Use Quantum-Safe Algorithms
Implementing post-quantum cryptography requires you to move away from traditional systems and adopt new, quantum-resistant algorithms. This transition involves a thorough understanding of various PQC methods and their appropriate usage in different scenarios.
Lattice-Based Cryptography
Lattice-based cryptography bases its security on the difficulty of solving certain problems in high-dimensional lattices. Even quantum computers find these problems challenging. Current implementations include:
1. CRYSTALS-Kyber
Selected by NIST for post-quantum key encapsulation
Offers strong security with reasonable key sizes
Efficient implementation possibilities
2. CRYSTALS-Dilithium
Digital signature scheme
Complements Kyber for complete post-quantum security
Hash-Based Signatures
Hash-based signatures offer another quantum-resistant approach. They rely only on the security of hash functions, making them a conservative choice for quantum resistance. Examples include:
1. SPHINCS+
Stateless hash-based signature scheme
Larger signatures but strong security guarantees
No need to maintain state between signatures
2. XMSS (eXtended Merkle Signature Scheme)
More efficient but requires state management
Suitable for controlled environments
Tools for Quantum-Safe Development
To implement quantum-safe cryptography, several tools are available:
1. Open Quantum Safe (OQS) Project
The Open Quantum Safe Project provides developers with the fundamental tools needed to implement quantum-resistant cryptography. Think of it as a comprehensive toolkit for transitioning existing systems to quantum-safe alternatives.
At the core is the liboqs C library, which provides essential building blocks for quantum-safe cryptography. This library matters because it:
Offers consistent interfaces across different quantum-resistant algorithms
Maintains compatibility with existing systems through OpenSSL integration
Provides thoroughly tested implementations of leading post-quantum algorithms
When implementing OQS in your projects, focus first on understanding its architecture and integration patterns. Most developers start with the OpenSSL integration, which allows for a gradual transition by supporting hybrid approaches - combining classical and quantum-resistant algorithms in the same system.
2. NIST Post-Quantum Cryptography Standardization
The NIST standardization program provides essential guidance for quantum-safe cryptography implementation.
Its implementation guidelines address three key areas: security level selection, algorithm choice, and performance optimization. Through these guidelines, developers can select appropriate security levels based on their application requirements and choose quantum-resistant algorithms that match their performance needs. NIST's guidelines also clarify the tradeoffs between different quantum-resistant approaches, helping developers make informed decisions for their systems.
The program's testing frameworks also serve an important role in ensuring implementation security.
These frameworks validate implementations against known test vectors, verify system interoperability, and identify potential vulnerabilities. This systematic testing approach helps maintain consistent security standards across quantum-safe implementations.
How Developers Can Adapt
Quantum computing advances require you to evaluate and update your cryptographic implementations. This process involves assessing current systems, identifying vulnerabilities, and implementing quantum-resistant solutions.
Understanding the practical steps and realistic timelines helps you plan an effective transition to quantum-safe security.
Implementation Steps
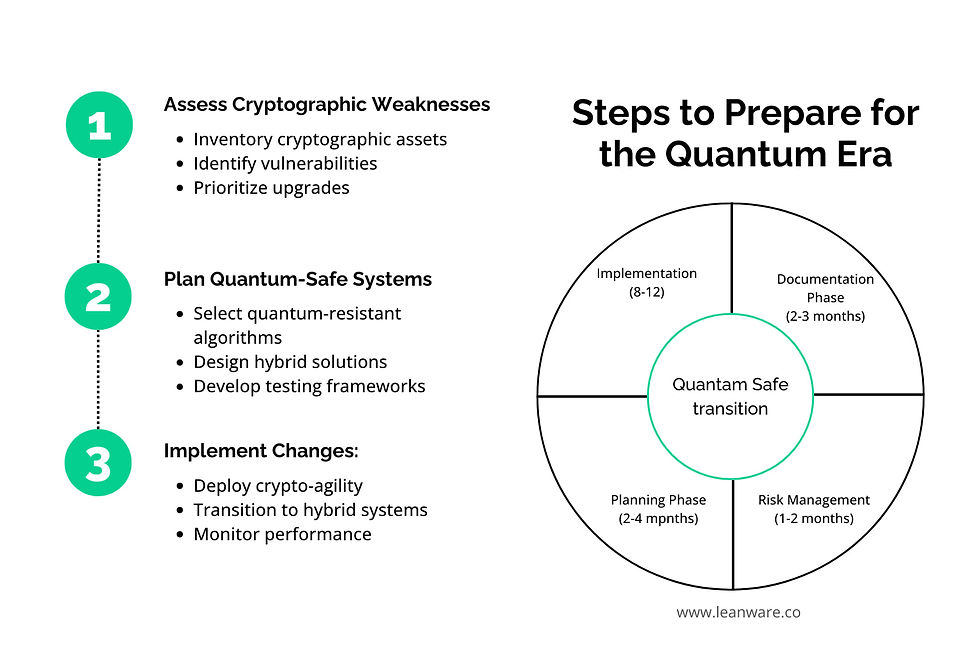
Finding Cryptographic Weaknesses
The process begins with a thorough assessment of your current cryptographic implementations. The documentation phase typically spans two to three months, during which development teams need to create a comprehensive inventory of their cryptographic assets.
This includes systematically cataloging every cryptographic operation in use, identifying the specific algorithms implemented across different systems, and creating detailed maps of data sensitivity levels. An important part of this phase involves documenting current key lengths and security parameters to identify potential vulnerabilities.
The subsequent risk assessment phase (months 1-2) evaluates vulnerabilities and planning needs:
Analyze systems against projected quantum threat timelines
Measure upgrade complexity and resource requirements
Calculate potential business impact of transitions
Create a prioritized system upgrade schedule
Switching to Quantum-Safe Systems
The planning phase (months 3-4) centers on designing quantum-safe solutions. Teams select appropriate quantum-resistant algorithms and develop hybrid approaches while establishing rollback procedures and testing frameworks.
During the implementation phase (months 6-12), teams deploy crypto-agility frameworks for flexible algorithm updates. This includes implementing hybrid solutions and monitoring performance to maintain system efficiency throughout the transition.
This systematic approach ensures a secure transition while maintaining current security standards and system stability.
Using Quantum-Inspired Algorithms
Quantum-inspired algorithms represent an important bridge between classical and quantum computing. These algorithms adapt key quantum principles - like examining multiple states simultaneously - to enhance traditional computing methods, allowing developers to solve complex problems more efficiently on today's hardware.
Optimization Applications
In optimization problems, quantum-inspired algorithms transform traditional approaches. For traffic routing, these algorithms evaluate multiple routes simultaneously rather than sequentially.
This same efficient parallel processing applies to resource allocation in data centers and portfolio optimization in finance, where analyzing numerous combinations at once leads to faster, more effective solutions.
Machine Learning Enhancement
These techniques bring significant improvements to machine learning model development through enhanced parallel processing. Here's how:
Feature selection becomes more efficient by evaluating multiple feature combinations simultaneously
Pattern recognition improves through parallel analysis of multiple patterns
Neural network training accelerates by exploring different architectures at once
This parallel processing approach significantly speeds up both development and training while maintaining high accuracy standards.
Impact on Startups and Existing Apps
When transitioning to quantum-safe computing, you need to balance immediate security needs with practical implementation challenges. This shift will affect both your system architecture and development practices.
Assessment and Planning
Start by evaluating your current infrastructure to identify systems that handle sensitive data and require early quantum-safe protection. This assessment helps you map critical dependencies and understand your transition scope clearly.
Team Development
Your success in quantum-safe implementation depends heavily on team preparation. Your development teams need focused training in quantum-safe principles and implementation practices. Building this expertise internally helps you make informed decisions about your security architecture.
Implementation Strategy
A phased implementation approach helps you manage transition risks effectively. You should:
Begin with non-critical systems to gain implementation experience
Adopt hybrid approaches that combine classical and quantum-safe methods
Monitor system performance to maintain operational efficiency
Staying Updated on Quantum Computing
Quantum computing advances affect software development practices, particularly in system security and algorithm implementation. Understanding these changes helps you prepare secure, future-ready applications.
Tracking Key Advances
Qubit development and error correction form the technical foundation for quantum computing progress. Current error rates and coherence times indicate quantum computers' ability to perform reliable computations.
When tracking developments, pay attention to how improved error correction brings us closer to practical quantum computation that could affect cryptographic security.
Building Community Connections
The quantum computing community provides specific resources for developers implementing quantum-safe solutions. Quantum Computing Stack Exchange offers practical implementation guidance, while quantum projects on GitHub demonstrate working examples of quantum-resistant algorithms. Professional networks through IEEE and ACM provide technical standards and implementation best practices.
Essential Skills Development
Quantum computing requires specific technical skills that build on software development fundamentals. Understanding quantum algorithms helps you identify potential vulnerabilities in current cryptographic implementations. Knowledge of quantum-resistant cryptography enables you to implement secure solutions in existing systems.
The Wrap
The quantum computing revolution isn't just about faster computers - it's about fundamentally different ways of solving problems. As software developers, we need to:
Understand quantum principles
Prepare our systems for quantum threats
Explore quantum-inspired solutions
Stay informed about advances
The future belongs to developers who can bridge classical and quantum computing, creating powerful, and future-proof applications. Start preparing today for tomorrow's quantum-enabled future.
Remember: The goal isn't to replace classical computing but to enhance our computational capabilities. By understanding and preparing for quantum computing now, we ensure our applications remain secure and effective in the quantum era.